Python: Playing With Numbers
- Pulkit Sahu
- Apr 3
- 3 min read
Python allows you to represent and work with integers, decimal, and complex numbers efficiently.
Let us see some numbers:
0 is a number invented by Aryabhatta.
3.14 is called a pi number, it is a decimal number.
10 is a whole number.
-1 is a negative number.
Now, how can we represent such numbers in Python?
Here, we have something new:
int
float
complex
The above classes are called numeric data types in Python.
Quick Links
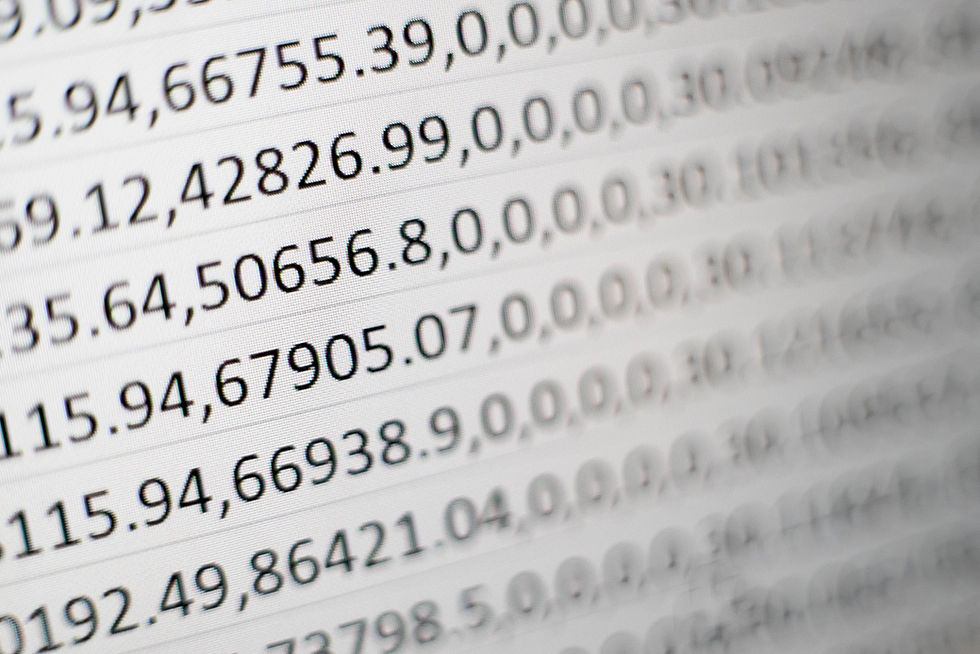
#1: Numbers in Python
int is a class name for integers (1, 10, 25),
float is a class name for decimal numbers (3.14, 0.05, 1.05), and complex denotes complex numbers.
We can use our previous knowledge of variables and assignment operator = to initiate a number in Python.
number = 0
Voila! You have successfully represented a number in Python. You can try here.
Let's break it down:
We're assigning 0 to number, where number is a variable name. Here, = is the assignment operator used to allot 0 to number. Now, 0 lives in number.
You don’t need to explicitly specify the data type (int or float). You can write:
pi = 3.14
You can find out the data type of a number using the type() function.
type(pi)
Let's print it:
print(type(pi))
# You'll get this:
<class 'float'>
Be careful about the parentheses:
The inner one is for the variable pi.
The outer one is for the print function.
#2: Can we play with these numbers in Python—add, subtract, multiply?
Yes, of course! These operations are allowed:
# Operators
+ - / % **
Add operator (+):
10 + 10
Subtract operator (-):
10 - 10
Multiply operator (*):
10 * 10
Division operator (/):
10 / 10
Modulus operator (%):
It gives the remainder of a division.
For example:
10 % 3 # Remainder is 1
Exponent operator (**):
2 ** 2
Floor division operator (//):
10 // 3 # It leaves the decimal part of the answer
You can run all of these codes here.
Tip:
You can use spaces between the operator and numbers for better readability.
Order of Operations
Remember, Python follows standard operator precedence (PEMDAS/BODMAS rules).
#3: Floats and Constants in Python
Floats are basically decimal point numbers in Python. For example, 20 / 3 returns a floating point number.
division = 20 / 3
print(division)
# Returns
6.666666666666667
To format floating-point numbers, we use f-strings or the format method. Inside the parentheses, we first write f before ".
print(f"")
Now, we use a placeholder (curly braces {}) to write the variable name division in it.
print(f"{division}")
A modifier is then used to modify its value (6.666666666666667) in the desired format. Here, we want to print the result up to two decimal places, modifier :.2f
print(f"{division:.2f}") # .2f represents upto two decimal places
# More examples
{95:.2f} or 95.00
n = 1000000
print(f"{n:,}") # for using commas as a separator, it gives
1,000,000
x = 10.2
y = 10
x = int(x)
y = float(y)
Read more here. The use of int(x) or float(x) is called "casting." It is used to convert a number or string from one data type to another.
print(x)
print(y)
We can also use Python's round() function to round a number. Just pass the variable name inside the parentheses of the round() function.
number = 10.2345
round(number)
# It gives 10
You can round a number upto a given decimal places. Just pass the number of decimal places as an argument after a comma.
round(number, 2) # for rounding off upto 2 decimal places.
# It gives 10.23
Constants
Variables whose value doesn't change while your program runs are referred as constants.
A popular example is 3.14 or pi. There is no built-in function or data type in Python for constants. You can make your constants by writing their names in upper case and assigning the fixed value.
PI = 3.14
In the next post, we will learn about a new structure to store great things for you.
References
Sources
Comments