Python: What's Your Name?
- Pulkit Sahu
- Mar 18
- 3 min read
Updated: May 12
Python variables are like boxes or containers where you store things like names, sentences, numbers, functions, and much more.
In the last post, we saw how to print "Hello, world" and other things. But writing print statements every time may not be the best idea. Why not store our stuff in containers called variables?
For example, writing or printing your name like this:
name = "Pulkit"
print(name)
Quick Links

#1: Your Name in Python
Remember, Python interpreter reads the code from top to bottom and from left to right.
Thus, on line 1, the word "Pulkit" is assigned to a variable called name. Now, "Pulkit" lives inside this new variable name.
On the second line, we can use our print statement with the name variable inside the parentheses. Notice that we're not quoting the name inside "" as it is a variable consisting of some value. This will give you: Pulkit.
#2: Common Python Variable Names
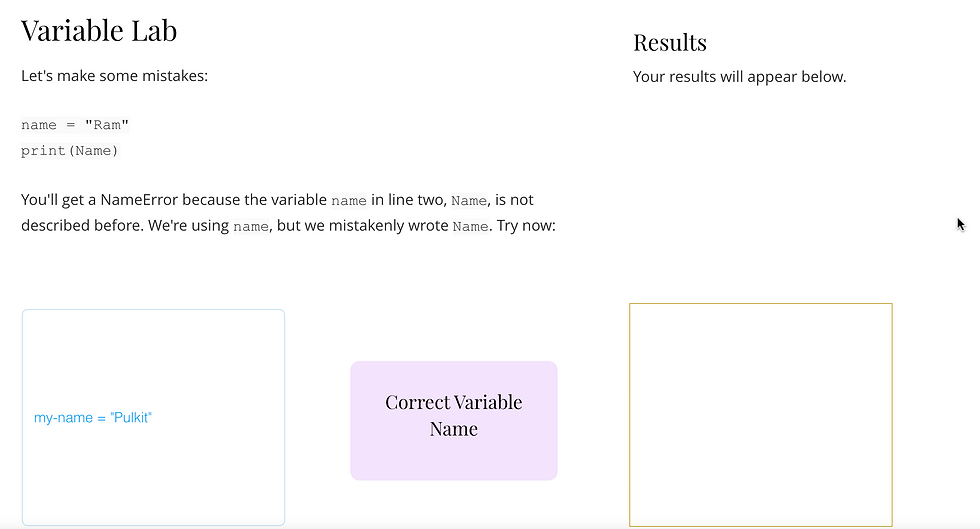
Some common rules for choosing variable names:
Variable names should make sense to you or your program readers. For example, a variable name like name indicates some name might have been assigned to it. But something like nm might confuse you or your readers. Rules:
Only numbers, alphabets, and underscores are allowed in variable names.
No spaces and no starting with numbers.
# Valid examples:
# Alphabets and numbers allowed
book1 = "Harry Potter and the Philosopher's Stone"
# An underscore and alphabets
my_name = "Hermione Granger"
# Invalid examples:
# Contains a space
book 2 = "Harry Potter and the Chamber of Secrets"
# Starts with a number, and "Python" is a keyword
3python = "Released in 2008"
No hyphens or special characters are allowed. Variable names can’t be the same as Python keywords.
# Invalid examples:
my-name = "Harry Potter" # hyphen is not allowed
name@ = "Ron" # special character @
for = "Always" # using Python keyword for
Variable names are case-sensitive. For example, these are four different variable names:
name = "Rama"
Name = "Sita"
NAME = "Lakshmana"
NaMe = "Ravana"
Comments are used to write useful things.
Comments are started with a hash or pound mark # in Python or with triple quotation marks """""". Comments follow a space or codes. # This is a comment.
#3: Multiple Python Variables with Styles
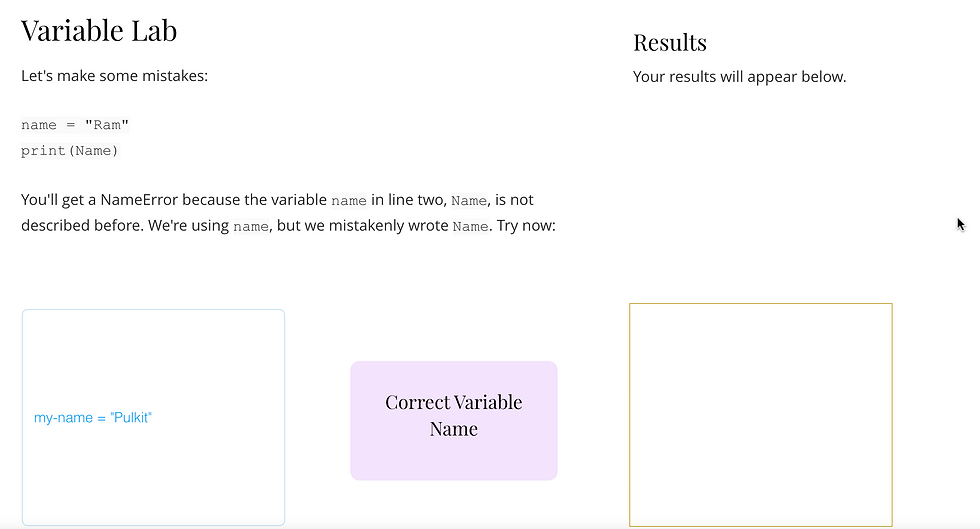
Multiple variables can be separated by commas:
In the code below, there are two variables on the left side, x and y, separated by a comma. We're assigning 10 and 20 (also separated by a comma) to x and y respectively.
x, y = 10, 20
You can check:
print(x)
print(y)
10
20
We can write long variable names in different styles:
Camel case
The starting letter of the first word is small, while the next words are capitalised.
nameOfVariable = "Vasco da Gama"
Pascal case
The first letter of each word is capitalised.
NameOfVariable = 10
Snake case
Words or characters are separated by underscores.
name_of_variable = 3.14
In the next post, we’ll see how variables allow us to do wonderful things in Python, along with functions.
References
Sources
Comments